#include <stdlib.h> int rand(void); |
rand() 함수
0부터 RAND_MAX(32767) 범위의 임의의 난수를 생성합니다.
이 함수는 정수를 생성합니다.
실수를 생성하는 방법은 아래쪽에 설명되어 있습니다.
rand() 함수를 사용하기 위해서는 다음 헤더를 포함해야 합니다.
#include <stdlib.h> |
#include <stdio.h>
#include <stdlib.h>
int main()
{
int i;
for (i = 0; i < 10; i++)
printf("%d\n", rand());
}
(Output)
41 18467 6334 26500 19169 15724 11478 29358 26962 24464 |
rand() 함수는 seed인 난수표에서 값을 가져오기 때문에 위 코드를 여러번 실행해도 언제나 같은 결과가 출력되는 것을 알 수 있습니다.
매번 다른 난수를 생성하기 위해서는 srand()함수를 이용해서 난수 생성기를 초기화해야 합니다.
srand() 함수
난수 생성기를 초기화시킵니다.
void srand(unsigned seed); |
rand() 함수는 기본으로 인수값이 1인 srand 함수를 초기화 시킵니다. seed를 이용해서 난수표의 새로운 시작점을 설정합니다.
다음 코드는 시스템의 시간을 이용해서 난수표를 초기화 하는 예입니다.
time_t t; srand((unsigned) time(&t)); |
srand((unsigned) time( NULL)); |
다음 코드를 이용해서 3번을 반복했습니다. 매번 다른 값이 출력되는 것을 알 수 있습니다.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int i;
srand((unsigned)time(NULL));
for (i = 0; i < 10; i++)
printf("%d\n", rand());
}
( Output)
10626 6903 30569 31440 25812 20171 19148 21324 1463 |
7489 778 4957 20494 30958 10659 31993 23620 21870 |
5921 30484 24919 15022 762 5903 5647 8385 32074 |
일정한 범위의 난수를 생성하는 방법
rand() 함수는 0 32767까지의 정수 난수를 생성합니다.
다음과 같은 방법을 이용해서 원하는 정수 범위의 난수를 생성할 수 있습니다.
rand() % 100; // 100으로 나눈 나머지이기 때문에 0 ~99까지의 난수를 생성합니다.
rand() % 101; // 101으로 나눈 나머지이기 때문에 0 ~100까지의 난수를 생성합니다.
rand() % 100 + 1; // 0 ~99까지의 범위에 1을 더하기 때문에 1 ~100 사이의 난수를 생성합니다 .
50부터 100까지의 난수를 생성하기 위해서는 다음과 같이 할 수 있습니다.
rand() % 51 + 50; // 0 ~50의 난수에 50을 더하기 때문에 50 ~100까지의 난수를 생성합니다.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int i;
srand((unsigned)time(NULL));
for (i = 0; i < 10; i++)
printf("%d\n", rand() % 100 + 1); // 1~100 사이의 난수
}
실수 범위의 난수를 생성하는 방법
예를 들어 0~1 사이의 실수 난수를 생성하기 위해서는 다음과 같은 식을 사용할 수 있습니다.
** rand()함수의 최대값이 32767임을 다시한 번 생각하기 바립니다.
(double)rand() / 100000; |
코드로 작성해 보겠습니다.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int i;
srand((unsigned)time(NULL));
for (i = 0; i < 10; i++)
printf("%f\n", ((double)((rand() % 10000))) / 10000);
}
소수 한 자리 숫자를 생성하기 위해서는 다음과 같이 할 수 있겠습니다.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int i;
srand((unsigned)time(NULL));
for (i = 0; i < 10; i++)
printf("%.1f\n", (rand() % 10) /10.0);
}
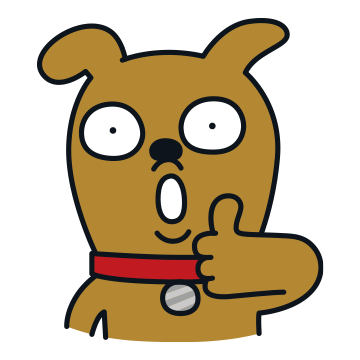
'C_C++ > C_라이브러리_함수' 카테고리의 다른 글
(C언어) strstr: 문자열에서 특정 문자열을 검색한다 (0) | 2022.11.21 |
---|---|
(C언어) 파일 복사: fgets(), fputs() 함수를 이용한 파일 복사 (0) | 2022.11.13 |
(C언어) sqrt() 함수: 양의 제곱근을 구한다 (0) | 2022.11.07 |
(C언어) pow, powl: x의 y제곱을 계산한다 (0) | 2022.11.06 |
(C언어) qsort 함수를 이용한 문자열 정렬하기 (0) | 2022.10.03 |