반응형
#학점구하기
0~100 사이의 점수를 입력받아서 90점 이상이면 "A", 80 이상이면 "B", 70이상이면 "C", 60 이상이면 "D", 60 미만이면 "F"를 출력하는 프로그램입니다.
if문을 사용해서 코딩했습니다.
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
int main()
{
int score;
char c;
while (1)
{
printf("점수(0~100): ");
scanf("%d", &score);
if (score < 0) break;
if (score >= 90) c = 'A';
else if (score >= 80) c = 'B';
else if (score >= 70) c = 'C';
else if (score >= 60) c = 'D';
else
c = 'F';
printf("점수: %d, 학점: %c\n", score, c);
}
return 0;
}
(Output)
90점 단위로 학점을 구하는 프로그램으로 수정했습니다.
학점을 구하는 부분은 함수로 처리했고, if문 대신에 switch~case 구문을 사용했습니다.
#define _CRT_SECURE_NO_WARNINGS
#include <stdio.h>
char* hakjum(int score)
{
char* h;
switch (score / 5)
{
case 20:
case 19: h = "A+"; break;
case 18: h = "A"; break;
case 17: h = "B+"; break;
case 16: h = "B"; break;
case 15: h = "C+"; break;
case 14: h = "C"; break;
case 13: h = "D+"; break;
case 12: h = "D"; break;
default:
h = "F";
break;
}
return h;
}
int main()
{
int i, score[10];
printf("점수를 10개 입력하세요: ");
for (i = 0; i < 10; i++)
scanf("%d", &score[i]);
for (i = 0; i < 10; i++)
printf("%3d: %s\n", score[i], hakjum(score[i]));
return 0;
}
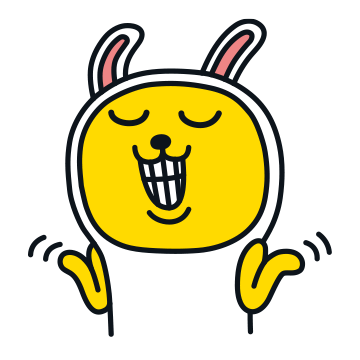
반응형
'C_C++' 카테고리의 다른 글
(C언어) 구구단 가로로 세로로 출력하기 (0) | 2022.10.24 |
---|---|
(C언어) 평균, 표준편차, 분산 구하기 (0) | 2022.10.24 |
(C언어) 10진수를 16진수로 변환: 배열 이용 (0) | 2022.10.23 |
(C언어) 10진수를 2진수로 변환: 배열 이용 (0) | 2022.10.23 |
10진수를 2진수로 표현하는 방법 (0) | 2022.10.22 |