반응형
C++에서 텍스트 파일을 저장하고 읽어오는 방법은 파일 입출력을 지원하는 <fstream> 헤더를 사용하여 구현할 수 있습니다. 아래는 텍스트 파일에 데이터를 저장하고 다시 읽어오는 예제입니다.
1. 텍스트 파일 저장하기 (쓰기)
파일에 데이터를 저장하려면 std::ofstream을 사용합니다.
#include <iostream>
#include <fstream> // 파일 입출력 헤더
int main() {
std::ofstream outFile("example.txt"); // 쓰기 모드로 파일 열기
if (!outFile) {
std::cerr << "파일을 열 수 없습니다!" << std::endl;
return 1;
}
// 파일에 데이터 쓰기
outFile << "안녕하세요, C++ 파일 입출력 예제입니다." << std::endl;
outFile << "두 번째 줄입니다." << std::endl;
outFile.close(); // 파일 닫기
std::cout << "파일 저장 완료!" << std::endl;
return 0;
}
2. 텍스트 파일 읽어오기
파일에서 데이터를 읽으려면 std::ifstream을 사용합니다.
#include <iostream>
#include <fstream> // 파일 입출력 헤더
#include <string> // 문자열 처리를 위한 헤더
int main() {
std::ifstream inFile("example.txt"); // 읽기 모드로 파일 열기
if (!inFile) {
std::cerr << "파일을 열 수 없습니다!" << std::endl;
return 1;
}
std::string line;
while (std::getline(inFile, line)) { // 한 줄씩 읽기
std::cout << line << std::endl;
}
inFile.close(); // 파일 닫기
return 0;
}
3. 주요 포인트
- 파일 경로: 위 코드에서는 파일 이름을 example.txt로 지정했지만, 절대 경로나 상대 경로를 지정할 수도 있습니다.
- 파일 모드:
- std::ofstream: 파일 쓰기 전용 (기존 파일을 덮어씀)
- std::ifstream: 파일 읽기 전용
- std::fstream: 읽기 및 쓰기 가능 (모드 설정 필요)
4. 읽기와 쓰기를 동시에 사용하는 예제
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::fstream file("example.txt", std::ios::in | std::ios::out | std::ios::app);
if (!file) {
std::cerr << "파일을 열 수 없습니다!" << std::endl;
return 1;
}
// 파일에 쓰기
file << "새로운 줄을 추가합니다." << std::endl;
// 파일 커서를 처음으로 이동
file.seekg(0, std::ios::beg);
// 파일 읽기
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
return 0;
}
위 코드를 실행하면, 기존 내용 끝에 새 내용을 추가한 뒤 파일 전체를 읽어 출력합니다.
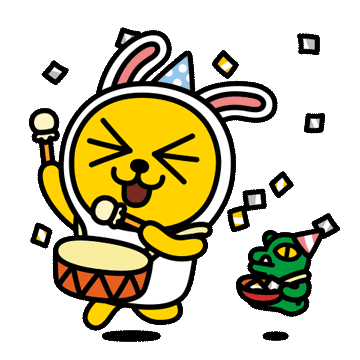
반응형
'C_C++' 카테고리의 다른 글
C++, 소수점 자릿수 지정, 오른쪽 정렬 줄맞추기 (0) | 2024.11.23 |
---|---|
C++, setw 출력 폭 지정하기 (0) | 2024.11.22 |
C++ 예외처리 Exception Handling (0) | 2024.11.20 |
C++, <vector> 템플릿 이용하기 (0) | 2024.11.19 |
C++, 클래스 템플릿 class template (0) | 2024.11.18 |